오늘은 데이트에 관해 알아보자.
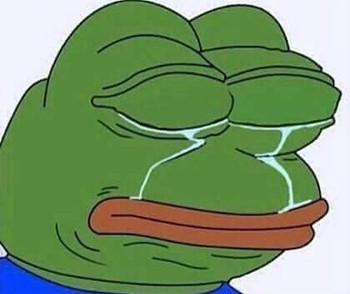
Date?
- Date가 무엇인지 우선 애플의 공식문서를 통해 알아보자.
https://developer.apple.com/documentation/foundation/date
간단하게 설명을 보면, 특정 시간을 기준으로 현재 시간까지 얼마나 떨어져있는 지를 알려준다.
이때 기준 시간은 UTC라는 국제 표준 시간을 사용한다.
따라서 정리해보면, 만약 Date 인스턴스를 생성하게 된다면, UTC(2001.01.01.00:00:00) 시를 기준으로 몇초후인지에 대한 시간 정보를 통해, 현재시점을 저장한다.
이는 암시적인 날짜, 시간으로 구성된다.
let now = Date() // "25 Feb 2024 at 1:15 PM"
print(now) // 2024-02-25 04:15:42 +0000
따라서, 제대로 사용하려면 달력 / 지역을 통해 변환해서 사용해야 한다.
필요한 경우엔, 적절한 형식(문자열)로 변환해서도 사용한다.
해당 파트는 사용 예시로만 거의 볼 예정이다.
그렇다면… 어떻게 적절하게 변환해서 사용해야 하는가?
Date 구조체를 활용하는 방법으론 다음과 같이 존재한다.
- Calendar 구조체
- 달력 및 달력의 요소를 다룬다.
- 년 / 월 / 일 + 시 / 분 / 초 + 요일
- ex: 몇살인지, 두 날짜 사이의 일수
- DateFormatter 클래스
- 형식 문자열로 변형해준다.
- 표시하기 위한 문자열로
- String 타입으로 적절하게 변환해준다.
그럼 아래서 살펴보자.
Calendar
Calendar | Apple Developer Documentation
- Date의 요소(년/월/일/시/분/초 등)로 분리가능하도록 만드는 구조체
var calendar = Calendar.current // 타입 속성 ==> 현재의 달력(양력) 반환
// gregorian (fixed) locale: en_001 time zone: Asia/Seoul (fixed) firstWeekday: 2 minDaysInFirstWeek: 1
let now = Date() // "25 Feb 2024 at 1:20 PM"
let year: Int = calendar.component(.year, from: now) // 2024
let month: Int = calendar.component(.month, from: now) // 2
let day: Int = calendar.component(.day, from: now) // 25
let timeHour: Int = calendar.component(.hour, from: now) // 13
let timeMinute: Int = calendar.component(.minute, from: now) // 20
let timeSecond: Int = calendar.component(.second, from: now) // 27
let weekday: Int = calendar.component(.weekday, from: now) // 1(일요일) ... 토요일은 7이다.
- 귀찮으면 한번에 얻는 방법도 있다.
let myCal = Calendar.current
var myDateCom = myCal.dateComponents([.year, .month, .day], from: now)
print("\(myDateCom.year!)년 \(myDateCom.month!)월 \(myDateCom.day!)일") // "2024년 2월 25일\n"
- 활용 예시
class Player {
var name: String
var yearOfBirth: Int
init(name: String, yearOfBirth: Int) {
self.name = name
self.yearOfBirth = yearOfBirth
}
var age: Int {
get {
let now = Date()
let year = Calendar.current.component(.year, from: now)
return year - yearOfBirth
}
}
}
let foden = Player(name: "포든", year: 2000)
foden.age
// 열거형으로 요일을 만들고, 오늘의 요일 계산
enum Weekday: Int {
case sunday = 1, monday, tuesday, wednesday, thursday, friday, saturday
// 타입 계산속성
static var today: Weekday {
let weekday: Int = Calendar.current.component(.weekday, from: Date())
return Weekday(rawValue: weekday)!
}
}
let today = Weekday.today
DateFormatter
DateFormatter | Apple Developer Documentation
- Date를 원하는 형식의 문자열로 쉽게 변환할 수 있는 클래스
우선 기억할 내용은 다음과 같다.
- Date를 특정형식의 문자열로 변환하려면
- ➞ (1)지역설정 + (2)시간대설정 + (3)날짜형식 + (4)시간형식
1. 나라 / 지역마다 날짜와 시간을 표시하는 형식과 언어가 다르기 때문에 필요한 경우 직접 설정해주어야 함.
let formatter = DateFormatter()
// 지역 및 시간대 설정
// (1) 지역 설정
formatter.locale = Locale(identifier: "ko_KR")
2. 시간대 설정(기본설정이 존재)
formatter.timeZone = TimeZone.current
3. 날짜, 시간 설정
// (1) 날짜 형식 선택
formatter.dateStyle = .full // "Sunday, February 25, 2024"
//formatter.dateStyle = .long // "February 25, 2024"
//formatter.dateStyle = .medium // "February 25, 2024"
//formatter.dateStyle = .none // (날짜 없어짐)
//formatter.dateStyle = .short // "2/25/24"
// (2) 시간 형식 선택
formatter.timeStyle = .full // "1:37:12 PM Korean Standard Time"
//formatter.timeStyle = .long // "1:37:12 PM GMT+9"
//formatter.timeStyle = .medium // "1:37:12 PM"
//formatter.timeStyle = .none // (시간 없어짐)
//formatter.timeStyle = .short // "1:37 PM"
// 실제 변환하기
let someString1 = formatter.string(from: Date()) // "Sunday, February 25, 2024 at 1:37:45 PM Korean Standard Time"
print(someString1)
// (3) 커스텀 형식으로 변환
formatter.dateFormat = "yyyy년 MMMM d일 (E)"
let someString2 = formatter.string(from: Date())
print(someString2) // "2024년 February 25일 (Sun)\n"
// (4) 문자열 -> Date
let newFormatter = DateFormatter()
newFormatter.dateFormat = "yyyy/MM/dd"
let someDate = newFormatter.date(from: "2024/02/25")!
print(someDate)
활용 예시
내가 아무것도 모르고 chatgpt로만.. SwiftUI에 대해 달력 부분을 맡은 적이 있어서 그때 활용한 코드를 다시 보았다.
[특정 영상을 참고했었는데 기억이 잘 나지 않는다.]
- 확장된 기능 사용을 위한 extension Date
- 날짜 구조체 선언
import SwiftUI
// 날짜 구조체
struct CalendarDate: Identifiable {
// id값
let id = UUID()
// 몇 일 e.g -> day: 27, day: 28 ...
var day: Int
// 전체 날짜 정보
var date: Date
// 이벤트 리스트
// -> var event: Event -> event Entity 만들어 질 시 optional 값으로 넣은 뒤
// --> 만약 nil 값이면 그대로, nil이 아니라면 달력 내 날짜 하단에 줄 표시
//var event: Int
}
struct CalendarPage: View {
// 요일 배열
let days = ["Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"]
// 선택된 월, 날짜를 관리하는 상태 속성
@State var selectedMonth = 0
@State var selectedDate = Date()
// 달력에서 선택되었는 지 아닌 지를 나타냄 -> 초깃값은 당일
@State private var onTapDate: Int? = Int(Date().getCurrentDay())
var body: some View {
VStack {
VStack {
// 달력 UI 생성하는 CalendarFunction
CalendarView()
// 해당 날짜의 계획된 세션을 ScrollView를 통해 조정
ScrollView {
EventList()
}
// Height를 우선적으로 screenHeight * 0.3으로 설정
.frame(maxHeight: screenHeight * 0.3)
Spacer()
}
// 화살표를 통해 월을 바꾸면 달력 내 모양도 바뀌는 것을 반영하기 위한 updateDate 함수
.onChange(of: selectedMonth) {
updateDate()
}
.ignoresSafeArea()
}
}
// 달력 UI 생성
@ViewBuilder
func CalendarView() -> some View {
VStack {
// 연도 출력
Text(selectedDate.printYear())
.foregroundStyle(Color.hex4E483C)
.fontWeight(.semibold)
.font(.system(size: 21))
.padding(.top, 60)
// 양 옆 화살표, 월 정보를 출력
HStack {
// '<' 버튼 -> 이전 월로 이동하는 버튼
Button {
selectedMonth -= 1
} label: {
Image(systemName: "arrowtriangle.left.fill")
.frame(width: 18, height: 18)
}
.foregroundStyle(Color.hex000000)
Spacer()
// 현재 월 표시
Text(selectedDate.printMonth())
.foregroundStyle(.hexB38DE2)
.fontWeight(.semibold)
.font(.system(size: 40))
Spacer()
// '>' 버튼 -> 다음 월로 이동하는 버튼
Button {
selectedMonth += 1
} label: {
Image(systemName: "arrowtriangle.right.fill")
.frame(width: 18, height: 18)
}
.foregroundStyle(Color.hex000000)
}
}
.padding(.horizontal, 36)
// 요일 출력
HStack {
// 반복문으로 요일 배열 내부 요소들 출력
ForEach(days, id:\.self) { day in
Text(day)
.foregroundStyle(Color.hex000000)
.font(.system(size: 14))
.frame(width: 40, height: 20)
}
}
.padding(.horizontal, 27)
// 일 출력
// LazyVGrid -> 수평 방향으로 나열된 그리드 레이아웃 생성하는 SwiftUI 컨테이너
// GridItem -> flexible 한 너비를 가진, count : 7개의 열로 된 그리드를 생성
LazyVGrid(columns: Array(repeating: GridItem(.flexible()), count: 7)) {
// fetchDates를 통해 나온 행에 맞춰 7개씩 출력
ForEach(fetchDates()){ value in
VStack {
if value.day != -1 {
// onTapDate : 클릭 된 게 아니라면 텍스트만 표시
if onTapDate != value.day {
Button {
onTapDate = value.day
} label: {
Text("\(value.day)")
}
}
// 클릭된 거라면 뒤에 원 표시
else {
Button {
onTapDate = value.day
} label: {
ZStack {
}
.background(
Circle()
.frame(width: 32, height: 32)
.foregroundStyle(Color.hex4E483C)
.overlay {
Text("\(value.day)")
.foregroundStyle(Color.hexFFFFFF)
Rectangle()
.foregroundStyle(Color.hexB38DE2)
.frame(width: 32, height: 3)
.position(x: 16.5, y: 40)
}
)
}
}
}
else {
// 빈 날짜의 경우 빈 텍스트로 표시
Text("")
}
}
.foregroundStyle(Color.hex4E483C)
.font(.system(size: 20))
.frame(width: 40, height: 46)
}
}
.padding(.horizontal, 32)
}
// 월 변경 시 날짜를 업데이트 해주는 함수
func updateDate() -> Void {
selectedDate = fetchSelectedMonth()
}
// 선택된 월의 날짜를 가져오는 함수
func fetchSelectedMonth() -> Date {
// Calendar.current를 통해 현재 사용 중인 달력을 얻음
let calendar = Calendar.current
// caldendar.date를 사용해 현재 날짜 + selectedMonth 만큼의 월을 더한 날짜를 얻음 , .month : 월을 더하는 단위
let month = calendar.date(byAdding: .month, value: selectedMonth, to: Date())
// date에서 옵셔널 'Date?' 를 반환하므로 강제 언래핑을 해줌 -> date에서 nil 이 반환되는 경우 에러 처리
return month!
}
// 선택된 월의 날짜를 가져오는 함수
func fetchDates() -> [CalendarDate] {
// 현재 달력을 사용하기 위해 현재 월을 가져옴
let calendar = Calendar.current
let currentMonth = fetchSelectedMonth()
// 현재 월에 속하는 날짜들을 가져와서 각 날짜를 CalendarDate로 매핑
// 즉, dates는 CalendarDate 구조체로 이루어진 배열
var dates: [CalendarDate] = currentMonth.datesOfMonth().map { date in
var calendarDate = CalendarDate(day: calendar.component(.day, from: date), date: date)
calendarDate.eventList = EventList() // 이 부분을 실제 EventList 객체로 대체
return calendarDate
}
// 현재 달의 첫 날이 무슨 요일인지 확인 -> (월요일, 1), (화요일, 2) ... 이런식으로 저장 -> 일요일 기준 시 "-1"부분 삭제
let firstDayOfWeek = calendar.component(.weekday, from: dates.first?.date ?? Date())
// 첫 주의 공백을 채우기 위해 빈 날짜를 -1로 추가
for _ in 0..<firstDayOfWeek - 1 {
dates.insert(CalendarDate(day: -1, date: Date()), at: 0)
}
// CaldendarDate(날짜) 배열 반환
return dates
}
}
// extension을 Date() 날짜 관련 기능 확장
extension Date {
// 연도 출력해주는 함수
func printYear() -> String {
// DateFormatter 를 사용하여 날짜를 특정 포맷의 문자열로 변환
let formatter = DateFormatter()
// 대문자 Y는 연도를 나타냄
formatter.dateFormat = "YYYY"
return formatter.string(from: self)
}
// 월 출력 함수
func printMonth() -> String {
let formatter = DateFormatter()
// 대문자 M은 월을 나타냄
formatter.dateFormat = "MM"
return formatter.string(from: self)
}
// 당일 가져오는 함수
func getCurrentDay() -> String {
let formatter = DateFormatter()
formatter.dateFormat = "dd"
return formatter.string(from: self)
}
// 해당 월의 모든 날짜를 반환해주는 함수
func datesOfMonth() -> [Date] {
// Calendar.current를 통해 현재 사용 중인 달력의 월, 연도를 얻음
let calendar = Calendar.current
let currentMonth = calendar.component(.month, from: self)
let currentYear = calendar.component(.year, from: self)
// DateComponents 함수를 통해 시작일의 연도와 월을 설정하고 날짜는 1로 설정
var startDateComponents = DateComponents()
startDateComponents.year = currentYear
startDateComponents.month = currentMonth
startDateComponents.day = 1
// startDate를 통해 startDateComponents에서 생성된 날짜를 얻음
let startDate = calendar.date(from: startDateComponents)!
// DateComponents 함수를 통해 종료일의 월을 1로 설정하고, 날짜를 -1로 설정
var endDateComponets = DateComponents()
endDateComponets.month = 1
endDateComponets.day = -1
// startDate + endDateComponents를 더하여 종료일을 얻음
let endDate = calendar.date(byAdding: endDateComponets, to: startDate)!
// Date 배열 생성
var dates: [Date] = []
var currentDate = startDate
// currentDate -> endDate 까지 해당 월에 속하는 모든 날짜가 포함됨
while currentDate <= endDate {
dates.append(currentDate)
currentDate = calendar.date(byAdding: .day, value: 1, to: currentDate)!
}
// 날짜
return dates
}
func string() -> String {
let formatter = DateFormatter()
formatter.dateFormat = "MM/dd/yyyy"
return formatter.string(from: self)
}
}
#Preview {
CalendarPage()
}
'iOS > Swift' 카테고리의 다른 글
About Class, Struct (1) | 2024.03.19 |
---|---|
About Delegate Pattern (0) | 2024.03.10 |
Result Type에 대한 이해 (1) | 2024.02.19 |
제네릭(Generics) (0) | 2024.02.18 |
비동기 프로그래밍(About Asynchronous) [2] (1) | 2024.02.17 |
오늘은 데이트에 관해 알아보자.
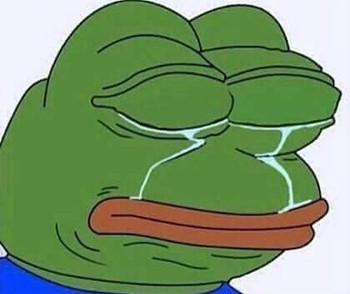
Date?
- Date가 무엇인지 우선 애플의 공식문서를 통해 알아보자.
https://developer.apple.com/documentation/foundation/date
간단하게 설명을 보면, 특정 시간을 기준으로 현재 시간까지 얼마나 떨어져있는 지를 알려준다.
이때 기준 시간은 UTC라는 국제 표준 시간을 사용한다.
따라서 정리해보면, 만약 Date 인스턴스를 생성하게 된다면, UTC(2001.01.01.00:00:00) 시를 기준으로 몇초후인지에 대한 시간 정보를 통해, 현재시점을 저장한다.
이는 암시적인 날짜, 시간으로 구성된다.
let now = Date() // "25 Feb 2024 at 1:15 PM"
print(now) // 2024-02-25 04:15:42 +0000
따라서, 제대로 사용하려면 달력 / 지역을 통해 변환해서 사용해야 한다.
필요한 경우엔, 적절한 형식(문자열)로 변환해서도 사용한다.
해당 파트는 사용 예시로만 거의 볼 예정이다.
그렇다면… 어떻게 적절하게 변환해서 사용해야 하는가?
Date 구조체를 활용하는 방법으론 다음과 같이 존재한다.
- Calendar 구조체
- 달력 및 달력의 요소를 다룬다.
- 년 / 월 / 일 + 시 / 분 / 초 + 요일
- ex: 몇살인지, 두 날짜 사이의 일수
- DateFormatter 클래스
- 형식 문자열로 변형해준다.
- 표시하기 위한 문자열로
- String 타입으로 적절하게 변환해준다.
그럼 아래서 살펴보자.
Calendar
Calendar | Apple Developer Documentation
- Date의 요소(년/월/일/시/분/초 등)로 분리가능하도록 만드는 구조체
var calendar = Calendar.current // 타입 속성 ==> 현재의 달력(양력) 반환
// gregorian (fixed) locale: en_001 time zone: Asia/Seoul (fixed) firstWeekday: 2 minDaysInFirstWeek: 1
let now = Date() // "25 Feb 2024 at 1:20 PM"
let year: Int = calendar.component(.year, from: now) // 2024
let month: Int = calendar.component(.month, from: now) // 2
let day: Int = calendar.component(.day, from: now) // 25
let timeHour: Int = calendar.component(.hour, from: now) // 13
let timeMinute: Int = calendar.component(.minute, from: now) // 20
let timeSecond: Int = calendar.component(.second, from: now) // 27
let weekday: Int = calendar.component(.weekday, from: now) // 1(일요일) ... 토요일은 7이다.
- 귀찮으면 한번에 얻는 방법도 있다.
let myCal = Calendar.current
var myDateCom = myCal.dateComponents([.year, .month, .day], from: now)
print("\(myDateCom.year!)년 \(myDateCom.month!)월 \(myDateCom.day!)일") // "2024년 2월 25일\n"
- 활용 예시
class Player {
var name: String
var yearOfBirth: Int
init(name: String, yearOfBirth: Int) {
self.name = name
self.yearOfBirth = yearOfBirth
}
var age: Int {
get {
let now = Date()
let year = Calendar.current.component(.year, from: now)
return year - yearOfBirth
}
}
}
let foden = Player(name: "포든", year: 2000)
foden.age
// 열거형으로 요일을 만들고, 오늘의 요일 계산
enum Weekday: Int {
case sunday = 1, monday, tuesday, wednesday, thursday, friday, saturday
// 타입 계산속성
static var today: Weekday {
let weekday: Int = Calendar.current.component(.weekday, from: Date())
return Weekday(rawValue: weekday)!
}
}
let today = Weekday.today
DateFormatter
DateFormatter | Apple Developer Documentation
- Date를 원하는 형식의 문자열로 쉽게 변환할 수 있는 클래스
우선 기억할 내용은 다음과 같다.
- Date를 특정형식의 문자열로 변환하려면
- ➞ (1)지역설정 + (2)시간대설정 + (3)날짜형식 + (4)시간형식
1. 나라 / 지역마다 날짜와 시간을 표시하는 형식과 언어가 다르기 때문에 필요한 경우 직접 설정해주어야 함.
let formatter = DateFormatter()
// 지역 및 시간대 설정
// (1) 지역 설정
formatter.locale = Locale(identifier: "ko_KR")
2. 시간대 설정(기본설정이 존재)
formatter.timeZone = TimeZone.current
3. 날짜, 시간 설정
// (1) 날짜 형식 선택
formatter.dateStyle = .full // "Sunday, February 25, 2024"
//formatter.dateStyle = .long // "February 25, 2024"
//formatter.dateStyle = .medium // "February 25, 2024"
//formatter.dateStyle = .none // (날짜 없어짐)
//formatter.dateStyle = .short // "2/25/24"
// (2) 시간 형식 선택
formatter.timeStyle = .full // "1:37:12 PM Korean Standard Time"
//formatter.timeStyle = .long // "1:37:12 PM GMT+9"
//formatter.timeStyle = .medium // "1:37:12 PM"
//formatter.timeStyle = .none // (시간 없어짐)
//formatter.timeStyle = .short // "1:37 PM"
// 실제 변환하기
let someString1 = formatter.string(from: Date()) // "Sunday, February 25, 2024 at 1:37:45 PM Korean Standard Time"
print(someString1)
// (3) 커스텀 형식으로 변환
formatter.dateFormat = "yyyy년 MMMM d일 (E)"
let someString2 = formatter.string(from: Date())
print(someString2) // "2024년 February 25일 (Sun)\n"
// (4) 문자열 -> Date
let newFormatter = DateFormatter()
newFormatter.dateFormat = "yyyy/MM/dd"
let someDate = newFormatter.date(from: "2024/02/25")!
print(someDate)
활용 예시
내가 아무것도 모르고 chatgpt로만.. SwiftUI에 대해 달력 부분을 맡은 적이 있어서 그때 활용한 코드를 다시 보았다.
[특정 영상을 참고했었는데 기억이 잘 나지 않는다.]
- 확장된 기능 사용을 위한 extension Date
- 날짜 구조체 선언
import SwiftUI
// 날짜 구조체
struct CalendarDate: Identifiable {
// id값
let id = UUID()
// 몇 일 e.g -> day: 27, day: 28 ...
var day: Int
// 전체 날짜 정보
var date: Date
// 이벤트 리스트
// -> var event: Event -> event Entity 만들어 질 시 optional 값으로 넣은 뒤
// --> 만약 nil 값이면 그대로, nil이 아니라면 달력 내 날짜 하단에 줄 표시
//var event: Int
}
struct CalendarPage: View {
// 요일 배열
let days = ["Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"]
// 선택된 월, 날짜를 관리하는 상태 속성
@State var selectedMonth = 0
@State var selectedDate = Date()
// 달력에서 선택되었는 지 아닌 지를 나타냄 -> 초깃값은 당일
@State private var onTapDate: Int? = Int(Date().getCurrentDay())
var body: some View {
VStack {
VStack {
// 달력 UI 생성하는 CalendarFunction
CalendarView()
// 해당 날짜의 계획된 세션을 ScrollView를 통해 조정
ScrollView {
EventList()
}
// Height를 우선적으로 screenHeight * 0.3으로 설정
.frame(maxHeight: screenHeight * 0.3)
Spacer()
}
// 화살표를 통해 월을 바꾸면 달력 내 모양도 바뀌는 것을 반영하기 위한 updateDate 함수
.onChange(of: selectedMonth) {
updateDate()
}
.ignoresSafeArea()
}
}
// 달력 UI 생성
@ViewBuilder
func CalendarView() -> some View {
VStack {
// 연도 출력
Text(selectedDate.printYear())
.foregroundStyle(Color.hex4E483C)
.fontWeight(.semibold)
.font(.system(size: 21))
.padding(.top, 60)
// 양 옆 화살표, 월 정보를 출력
HStack {
// '<' 버튼 -> 이전 월로 이동하는 버튼
Button {
selectedMonth -= 1
} label: {
Image(systemName: "arrowtriangle.left.fill")
.frame(width: 18, height: 18)
}
.foregroundStyle(Color.hex000000)
Spacer()
// 현재 월 표시
Text(selectedDate.printMonth())
.foregroundStyle(.hexB38DE2)
.fontWeight(.semibold)
.font(.system(size: 40))
Spacer()
// '>' 버튼 -> 다음 월로 이동하는 버튼
Button {
selectedMonth += 1
} label: {
Image(systemName: "arrowtriangle.right.fill")
.frame(width: 18, height: 18)
}
.foregroundStyle(Color.hex000000)
}
}
.padding(.horizontal, 36)
// 요일 출력
HStack {
// 반복문으로 요일 배열 내부 요소들 출력
ForEach(days, id:\.self) { day in
Text(day)
.foregroundStyle(Color.hex000000)
.font(.system(size: 14))
.frame(width: 40, height: 20)
}
}
.padding(.horizontal, 27)
// 일 출력
// LazyVGrid -> 수평 방향으로 나열된 그리드 레이아웃 생성하는 SwiftUI 컨테이너
// GridItem -> flexible 한 너비를 가진, count : 7개의 열로 된 그리드를 생성
LazyVGrid(columns: Array(repeating: GridItem(.flexible()), count: 7)) {
// fetchDates를 통해 나온 행에 맞춰 7개씩 출력
ForEach(fetchDates()){ value in
VStack {
if value.day != -1 {
// onTapDate : 클릭 된 게 아니라면 텍스트만 표시
if onTapDate != value.day {
Button {
onTapDate = value.day
} label: {
Text("\(value.day)")
}
}
// 클릭된 거라면 뒤에 원 표시
else {
Button {
onTapDate = value.day
} label: {
ZStack {
}
.background(
Circle()
.frame(width: 32, height: 32)
.foregroundStyle(Color.hex4E483C)
.overlay {
Text("\(value.day)")
.foregroundStyle(Color.hexFFFFFF)
Rectangle()
.foregroundStyle(Color.hexB38DE2)
.frame(width: 32, height: 3)
.position(x: 16.5, y: 40)
}
)
}
}
}
else {
// 빈 날짜의 경우 빈 텍스트로 표시
Text("")
}
}
.foregroundStyle(Color.hex4E483C)
.font(.system(size: 20))
.frame(width: 40, height: 46)
}
}
.padding(.horizontal, 32)
}
// 월 변경 시 날짜를 업데이트 해주는 함수
func updateDate() -> Void {
selectedDate = fetchSelectedMonth()
}
// 선택된 월의 날짜를 가져오는 함수
func fetchSelectedMonth() -> Date {
// Calendar.current를 통해 현재 사용 중인 달력을 얻음
let calendar = Calendar.current
// caldendar.date를 사용해 현재 날짜 + selectedMonth 만큼의 월을 더한 날짜를 얻음 , .month : 월을 더하는 단위
let month = calendar.date(byAdding: .month, value: selectedMonth, to: Date())
// date에서 옵셔널 'Date?' 를 반환하므로 강제 언래핑을 해줌 -> date에서 nil 이 반환되는 경우 에러 처리
return month!
}
// 선택된 월의 날짜를 가져오는 함수
func fetchDates() -> [CalendarDate] {
// 현재 달력을 사용하기 위해 현재 월을 가져옴
let calendar = Calendar.current
let currentMonth = fetchSelectedMonth()
// 현재 월에 속하는 날짜들을 가져와서 각 날짜를 CalendarDate로 매핑
// 즉, dates는 CalendarDate 구조체로 이루어진 배열
var dates: [CalendarDate] = currentMonth.datesOfMonth().map { date in
var calendarDate = CalendarDate(day: calendar.component(.day, from: date), date: date)
calendarDate.eventList = EventList() // 이 부분을 실제 EventList 객체로 대체
return calendarDate
}
// 현재 달의 첫 날이 무슨 요일인지 확인 -> (월요일, 1), (화요일, 2) ... 이런식으로 저장 -> 일요일 기준 시 "-1"부분 삭제
let firstDayOfWeek = calendar.component(.weekday, from: dates.first?.date ?? Date())
// 첫 주의 공백을 채우기 위해 빈 날짜를 -1로 추가
for _ in 0..<firstDayOfWeek - 1 {
dates.insert(CalendarDate(day: -1, date: Date()), at: 0)
}
// CaldendarDate(날짜) 배열 반환
return dates
}
}
// extension을 Date() 날짜 관련 기능 확장
extension Date {
// 연도 출력해주는 함수
func printYear() -> String {
// DateFormatter 를 사용하여 날짜를 특정 포맷의 문자열로 변환
let formatter = DateFormatter()
// 대문자 Y는 연도를 나타냄
formatter.dateFormat = "YYYY"
return formatter.string(from: self)
}
// 월 출력 함수
func printMonth() -> String {
let formatter = DateFormatter()
// 대문자 M은 월을 나타냄
formatter.dateFormat = "MM"
return formatter.string(from: self)
}
// 당일 가져오는 함수
func getCurrentDay() -> String {
let formatter = DateFormatter()
formatter.dateFormat = "dd"
return formatter.string(from: self)
}
// 해당 월의 모든 날짜를 반환해주는 함수
func datesOfMonth() -> [Date] {
// Calendar.current를 통해 현재 사용 중인 달력의 월, 연도를 얻음
let calendar = Calendar.current
let currentMonth = calendar.component(.month, from: self)
let currentYear = calendar.component(.year, from: self)
// DateComponents 함수를 통해 시작일의 연도와 월을 설정하고 날짜는 1로 설정
var startDateComponents = DateComponents()
startDateComponents.year = currentYear
startDateComponents.month = currentMonth
startDateComponents.day = 1
// startDate를 통해 startDateComponents에서 생성된 날짜를 얻음
let startDate = calendar.date(from: startDateComponents)!
// DateComponents 함수를 통해 종료일의 월을 1로 설정하고, 날짜를 -1로 설정
var endDateComponets = DateComponents()
endDateComponets.month = 1
endDateComponets.day = -1
// startDate + endDateComponents를 더하여 종료일을 얻음
let endDate = calendar.date(byAdding: endDateComponets, to: startDate)!
// Date 배열 생성
var dates: [Date] = []
var currentDate = startDate
// currentDate -> endDate 까지 해당 월에 속하는 모든 날짜가 포함됨
while currentDate <= endDate {
dates.append(currentDate)
currentDate = calendar.date(byAdding: .day, value: 1, to: currentDate)!
}
// 날짜
return dates
}
func string() -> String {
let formatter = DateFormatter()
formatter.dateFormat = "MM/dd/yyyy"
return formatter.string(from: self)
}
}
#Preview {
CalendarPage()
}
'iOS > Swift' 카테고리의 다른 글
About Class, Struct (1) | 2024.03.19 |
---|---|
About Delegate Pattern (0) | 2024.03.10 |
Result Type에 대한 이해 (1) | 2024.02.19 |
제네릭(Generics) (0) | 2024.02.18 |
비동기 프로그래밍(About Asynchronous) [2] (1) | 2024.02.17 |